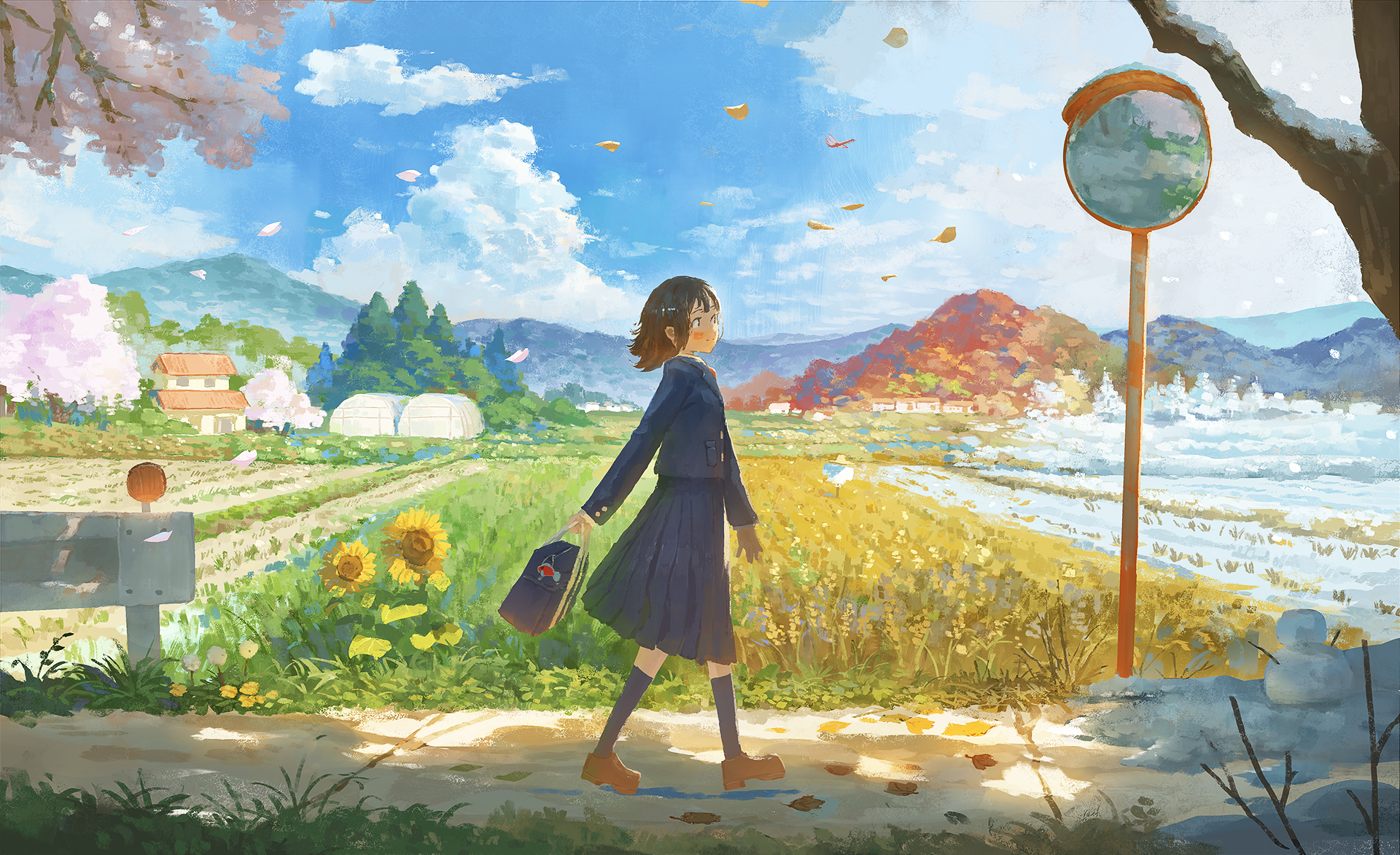
Java8 Stream流
AI-摘要
GPT
AI初始化中...
介绍自己
生成本文简介
推荐相关文章
前往主页
前往tianli博客
引言:
Java 8 引入的 Stream API 为集合数据的处理提供了一种全新的函数式编程方式。它允许开发者以声明式的方式对集合执行一系列中间操作和终止操作,从而大大简化代码逻辑。本文将系统介绍 Stream 的基本概念、使用步骤以及常见的操作模式,并通过代码示例展示如何使用流来高效处理数据。
流式操作简介 :
流(Stream) 是一种无需存储数据的计算视图,具备以下特点:
按需计算:流不存储数据,而是当需要时进行计算。
不可变性:流不会修改原有数据,而是返回一个新集合。
一次性使用:流只能消费一次,不能重复使用。
支持并行:可以通过
stream.parallel()
或parallelStream()
在多个线程中并行执行操作。
处理步骤 :
中间操作返回一个新的流,这些操作包括但不限于:
filter:过滤元素,接受一个返回
boolean
的函数。map:将每个元素映射成一个新的元素,创建新的数据“版本”。
flatMap:扁平化流,将多个流合并为一个流。
sorted:对流进行排序。 - distinct:去除重复元素。
peek:对每个元素执行操作并返回一个新的流,用于调试或日志输出。
skip:跳过前面若干个元素。
List<Stream<String>> collect1 = str.stream()
.map(d -> d.split(""))
.map(Arrays::stream)
.distinct()
.collect(Collectors.toList());
List<String> collect2 = str.stream()
.map(d -> d.split(""))
.flatMap(Arrays::stream)
.distinct()
.collect(Collectors.toList());
public class Main {
public static void main(String[] args) {
List<List<String>> listOfLists = Arrays.asList(
Arrays.asList("a", "b"),
Arrays.asList("c", "d")
);
List<String> flatList = listOfLists.stream()
.flatMap(List::stream) // 将每个子列表转换为流并展平
.collect(Collectors.toList());
System.out.println(flatList); // 输出: [a, b, c, d]
}
}
转换方式
map:一对一转换,每个元素映射为一个新元素。
flatMap:一对多转换,每个元素映射为一个流,最终展平为单一流。
输出流的大小
map:输出流的元素数量与输入流相同。
flatMap:输出流的元素数量取决于每个元素生成的流中元素的总数,可能与输入流不同。
处理嵌套结构
map:无法展平嵌套结构,会将嵌套结构作为单个元素处理。
flatMap:可以将嵌套结构展平,将嵌套元素提取到顶层流中。
// 对流中元素进行拼接
String shortMenu = menu.stream()
.map(Dish::getName)
.collect(Collectors.joining(", "));
* map还有一些变形的形式,例如:当需要对int类型的数据进行求和或者找最大最小值或者求平均值一般是先map()得到Stream流 然后再reduce(Integer::sum)等操作得到一个Optional对象 例如
* int calories=menu.stream().map(Dish::getCalories).reduce(0, Integer::sum); 可以改成
* int calories=menu.stream().mapToInt(Dish::getCalories).sum(); 即不用进行多于的拆箱操作而且写法更加简洁。
终止操作:
终止操作会触发流的计算,生成最终结果。常见的终止操作有:
forEach:对每个元素执行操作。
collect:将流转换为集合或其他数据结构。
reduce:归约操作,用于统计、求和、查找最大值或最小值。
toArray:将流转化成数组。
规约操作(Reduction):
使用 reduce 进行归约:适合对数值进行求和、求最值、求平均等操作。
int totalCalories = menu.stream()
.collect(Collectors.reducing(0, Dish::getCalories, Integer::sum));
IntSummaryStatistics menuStatistics = menu.stream()
.collect(Collectors.summarizingInt(Dish::getCalories));
// 输出示例:IntSummaryStatistics{count=9, sum=4300, min=120, average=477.78, max=800}
// Dish::getName 是一个方法引用的示例,属于java8中lambda表达式中的一种简化语法 (Dish::getName是指引用了Dish类中的getName()实例方法 其可以等价为dish->dish.getName)
int totalCalories=menu.stream().collect(reducing( 0, Dish::getCalories, (i, j)-> i+j));
第一个参数是归约操作的起始值,也是流中没有元素时的返回值,所以很显然对于数值和而言0是一个合适的值。
第二个参数就是你想要对哪个元素使用的函数,将菜肴转换成一个表示其所含热量的int。
第三个参数是一个BinaryOperator,将两个项目累积成一个同类型的值。这里它就是对两个int求和。
# reduce()与collect(reducing())代码解释:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// 带初始值的 reduce,计算总和
int sumWithIdentity = numbers.stream().reduce(0, Integer::sum); // 返回 15
// 不带初始值的 reduce,计算总和
Optional<Integer> sumWithoutIdentity = numbers.stream().reduce(Integer::sum);
Optional<Dish> mostCalorieDish= menu.stream().collect(reducing( (d1, d2)-> d1.getCalories() > d2.getCalories() ? d1 : d2));
查找最大值等价于:
Comparator<Dish> dishCaloriesComparator= Comparator.comparingInt(Dish::getCalories); Optional<Dish> mostCalorieDish= menu.stream() .collect(maxBy(dishCaloriesComparator));
# 分组操作
public enum CaloricLevel { DIET, NORMAL, FAT }
Map<CaloricLevel, List<Dish>> dishesByCaloricLevel = menu.stream()
.collect(Collectors.groupingBy(dish -> {
if (dish.getCalories() <= 400) return CaloricLevel.DIET;
else if (dish.getCalories() <= 700) return CaloricLevel.NORMAL;
else return CaloricLevel.FAT;
}));
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 小王
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果